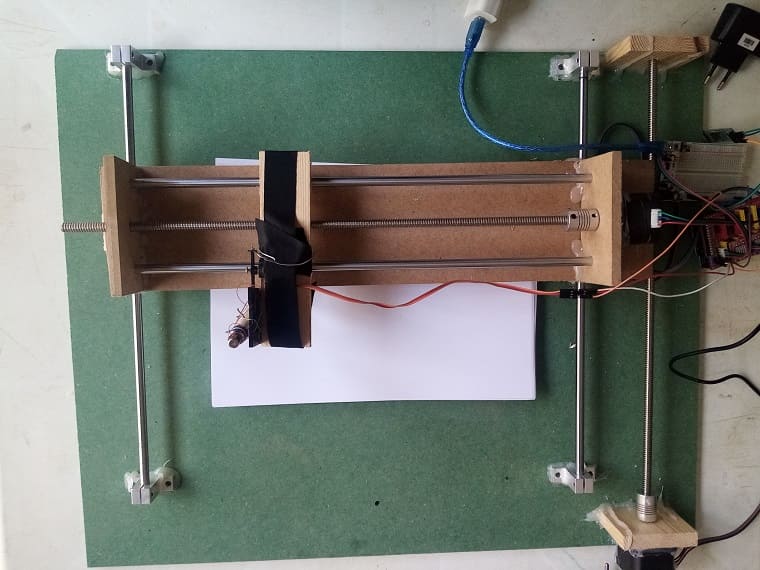
A printer is an electronic device that produces a printed image or text on paper or other media. There are several types of printers available, each with their own unique characteristics and advantages. The most common types are:
It is possible to create a DIY printer using an Arduino microcontroller and various other components. The specific components and design will depend on the type of printer you want to create and the features you want to include.
One popular approach for a DIY printer is to use a stepper motor to control the movement of the print head, and an inkjet print head or a thermal print head to create the printed image. The Arduino can be used to control the stepper motor and send commands to the print head to create the image.
To create a DIY printer, you will need to have a good understanding of electronics and programming, as well as the ability to design and assemble the mechanical components of the printer. Some of the necessary components for a basic DIY printer are:
US-17H4401 Stepper motor
Motor driver
Print head (inkjet or thermal)
Power supply
Mechanical parts (such as gears, pulleys, and belts)
An Arduino microcontroller can be used to control a DIY printer, including sending commands over Bluetooth to control the movement of the printer’s components. To do this, a Bluetooth module, such as the HC-05 or HC-06, can be connected to the Arduino. The Arduino can then be programmed to send commands to the Bluetooth module, which in turn sends commands to the stepper motor drivers controlling the movement of the printer‘s components.
The specific details of setting up an Arduino-controlled printer with Bluetooth functionality will depend on the design of the printer and the specific components being used. It may require some knowledge of programming and electronics to properly set it up.
In this project we will build a printer receiving the data to be printed by Bluetooth.
This printer is controlled by the Arduino UNO board through the CNC Shield board.
It also contains two stepper motors responsible for moving the pen along both axes (x) and (y) and a servo motor which raises or lowers the pen.
In addition, this printer uses the HC-06 Bluetooth module to receive the text to be printed by the Smartphone.
Arduino UNO
The Arduino UNO is a microcontroller board based on the ATmega328P microcontroller. It is one of the most popular boards in the Arduino family and is widely used for a wide range of projects and applications.
The Arduino UNO has several features that make it an ideal choice for many projects:
The Arduino UNO can be used for a wide variety of projects and applications, such as controlling lights and motors, reading sensors, communicating with other devices, and creating interactive projects.
Bluetooth module HC-06
The HC-06 is a Bluetooth module that can be used to add wireless communication capabilities to a device, such as a printer controlled by an Arduino. It is a slave module, which means that it can only connect to other devices and cannot initiate connections on its own. It can be connected to the Arduino using a serial connection and can be configured to work with a variety of baud rates, from 9600 to 115200.
CNC SHIELD
A CNC shield is a board that sits on top of an Arduino microcontroller and provides the necessary connections to control stepper motors in a CNC (computer numerical control) machine. The shield typically includes connectors for attaching stepper motor drivers and a power supply, as well as input pins for connecting limit switches and other devices.
CNC shields are designed to work with firmware such as GRBL, Marlin, etc. These firmware will allow the user to send G-code commands to the CNC machine. These commands can be sent to the machine via USB, serial or wireless communication, the shield will take care of converting those commands into the necessary step and direction signals to control the stepper motors.
The most common type of CNC shield is based on the Arduino UNO, it can control up to 4 stepper motors at once and can be used to control 3-axis CNC machines. Some CNC shield also includes an on-board spindle control, that can be used to control a spindle or laser diode.
two US-17H4401 Stepper motors
The US-17H4401 stepper motor is a type of motor that is commonly used in DIY printers. It is a hybrid stepper motor, which means that it combines the best features of both permanent magnet and variable reluctance stepper motors. This type of motor is known for its high holding torque, accuracy, and smooth movement. It can be controlled by a stepper motor driver and connected to a microcontroller or computer to control the movement of the printer’s print head and other components.
motor servo
A motor servo is a type of actuator that uses a closed-loop control system to control the position of a shaft or other mechanical component. It typically consists of a motor, a position sensor (such as a potentiometer), and control electronics. The control electronics use the position feedback from the sensor to adjust the motor’s power and direction in order to move the shaft to the desired position. Servo motors are commonly used in robotics, automation, and control systems.
CNC rod kit
A CNC rod kit is a set of components used to build or upgrade a CNC (computer numerical control) machine. The kit typically includes the linear rods that form the machine’s structure, as well as other components such as linear bearings, lead screws, and stepper motors. CNC machines are used for a wide range of manufacturing and prototyping applications, such as cutting, milling, and drilling, and can be used to make precise parts from a variety of materials. CNC rod kits are often used by hobbyists and DIY enthusiasts to build custom CNC machines or upgrade existing machines.
pen
First, you have to connect the Arduino board to the CNC board. Then, we connect the two stepper motors to the CNC board.
For the servo motor we connect:
the red wire to the 5v pin of the CNC board
the black file to GND of the CNC board
the yellow file to pin A4 of the CNC board
For the HC-06 bluetooth module we connect:
the VCC pin to 5V of the power supply module
the GND to GND pin of the CNC board
the TXD pin to the CNC board RX pin
the RXD pin to the TX pin of the CNC board
Here is the program that allows you to connect the Arduino card to the smartphone and receive messages by bluetooth in order to print it on paper.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 |
#include "printer.h" #include <SoftwareSerial.h> SoftwareSerial hc06(0,1); Servo myservo; // create servo object to control a servo String mot=""; void setup() { //Serial.begin(9600); hc06.begin(9600); myservo.attach(A4); pinMode(enPin, OUTPUT); digitalWrite(enPin, LOW); pinMode(stepPin, OUTPUT); pinMode(dirPin, OUTPUT); pinMode(stepPinx, OUTPUT); pinMode(dirPinx, OUTPUT); //Serial.println(F("CNC Shield Initialized")); //draw_9(myservo); myservo.write(120); delay(1000); // translationX(LOW,HIGH,5000); } void loop() { while (hc06.available()>0){ //Serial.write(hc06.read()); mot+=(char)hc06.read(); // receive data from bluetooth HC-06 //draw_espace(myservo); } if(mot!=""){ // Serial.println(strcmp(mot[0],'a')); for(int i =0; i < mot.length(); i++ ) { // Serial.println(mot[i]); if (mot[i]=='a') {draw_a(myservo); }// print the letter a if (mot[i]=='b') draw_b(myservo); // print the letter b if (mot[i]=='c') draw_c(myservo); if (mot[i]=='d') draw_d(myservo); if (mot[i]=='e') draw_e(myservo); if (mot[i]=='f') draw_f(myservo); if (mot[i]=='g') draw_g(myservo); if (mot[i]=='h') draw_h(myservo); if (mot[i]=='i') draw_i(myservo); if (mot[i]=='j') draw_j(myservo); if (mot[i]=='k') draw_k(myservo); if (mot[i]=='l') draw_l(myservo); if (mot[i]=='m') draw_m(myservo); if (mot[i]=='n') draw_n(myservo); if (mot[i]=='o') draw_o(myservo); if (mot[i]=='p') draw_p(myservo); if (mot[i]=='q') draw_q(myservo); if (mot[i]=='r') draw_r(myservo); if (mot[i]=='s') draw_s(myservo); if (mot[i]=='t') draw_t(myservo); if (mot[i]=='u') draw_u(myservo); if (mot[i]=='v') draw_v(myservo); if (mot[i]=='w') draw_w(myservo); if (mot[i]=='x') draw_x(myservo); if (mot[i]=='y') draw_y(myservo); if (mot[i]=='z') draw_z(myservo); if (mot[i]=='0') draw_0(myservo); if (mot[i]=='1') draw_1(myservo); if (mot[i]=='2') draw_2(myservo); if (mot[i]=='3') draw_3(myservo); if (mot[i]=='4') draw_4(myservo); if (mot[i]=='5') draw_6(myservo); if (mot[i]=='6') draw_6(myservo); if (mot[i]=='7') draw_7(myservo); if (mot[i]=='8') draw_8(myservo); if (mot[i]=='9') draw_9(myservo); if (mot[i]==' ') draw_espace(myservo); } mot=""; } delay(100); } |
On va créer une application mobile nommée ‘message_arduino’ avec App Inventor qui permet d’envoyer un message du smartphone à la carte Arduino.
On vous propose donc de réaliser le design de l’application, avec le visuel suivant:
Pour programmer l’application, App Inventor nous propose d’utiliser L’espace Blocs qui permet de créer un programme sous forme de schéma bloc. Très simple d’utilisation mais nécessitant un peu de logique de programmation.
Voici le programme de l’application réalisée dans l’espace Blocs de l’App Inventor:
เครดิตฟรี 28-01-2424
I'm curious to find out what blog platform you are using? I'm having some minor security issues with my latest blog and I would like to find something more safeguarded. Do you have any solutions?
บาคาร่า 12-11-2323
Hi to every one, since I am genuinely eager of reading this webpage's post to be updated daily. It includes nice stuff.
สมัครคาสิโนออนไลน์ 12-01-2323
After checking out a number of the blog posts on your site, I really like your technique of blogging. I saved as a favorite it to my bookmark site list and will be checking back in the near future. Please visit my web site too and tell me how you feel.
เครดิตฟรี 300 ถอนได้ 12-01-2323
It's remarkable to pay a quick visit this web site and reading the views of all colleagues concerning this post, while I am also zealous of getting knowledge.
สล็อตออนไลน์ สมาชิกใหม่รับเครดิตฟรี 100 12-01-2323
Greetings! Very useful advice within this post! It is the little changes that produce the greatest changes. Thanks a lot for sharing!
บาคาร่า1688 12-01-2323
This blog was... how do you say it? Relevant!! Finally I have found something that helped me. Cheers!
918kissเครดิตฟรี 12-01-2323
Excellent post. I was checking continuously this blog and I'm impressed! Very helpful info specially the last part :) I care for such info much. I was looking for this particular info for a long time. Thank you and good luck.
บาคาร่า ขั้นต่ำ 5 บาท 12-01-2323
Thanks for ones marvelous posting! I actually enjoyed reading it, you're a great author. I will be sure to bookmark your blog and may come back down the road. I want to encourage one to continue your great writing, have a nice weekend!
เครดิตฟรี ถอนง่าย 12-01-2323
If some one wishes expert view about blogging and site-building after that i suggest him/her to visit this web site, Keep up the nice job.
เครดิตฟรี pg 12-01-2323
Greetings! I know this is kinda off topic but I was wondering which blog platform are you using for this website? I'm getting sick and tired of Wordpress because I've had problems with hackers and I'm looking at options for another platform. I would be great if you could point me in the direction of a good platform.
แทง บาคาร่า 12-01-2323
Hi there I am so thrilled I found your web site, I really found you by accident, while I was searching on Yahoo for something else, Nonetheless I am here now and would just like to say thanks a lot for a marvelous post and a all round entertaining blog (I also love the theme/design), I don't have time to look over it all at the minute but I have book-marked it and also added in your RSS feeds, so when I have time I will be back to read much more, Please do keep up the fantastic work.