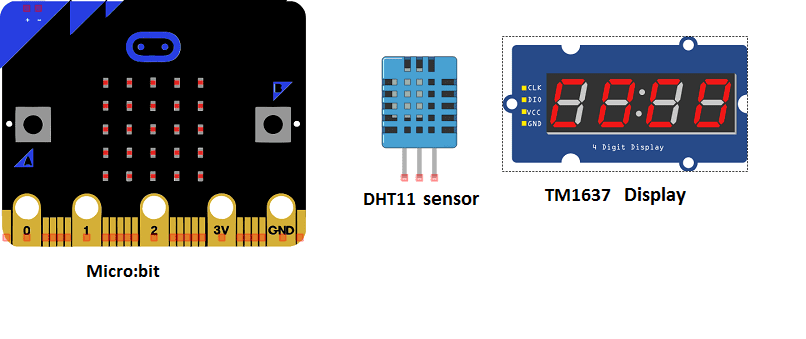
Measuring temperature with Arduino is a common application that can be achieved by using a temperature sensor such as the LM35 or the DS18B20. These sensors can be connected to an Arduino board and used to read the temperature and convert it into a digital value that can be read by the microcontroller.
The basic steps to measure temperature with Arduino are:
It’s important to check the datasheet of the sensor you’re using to have the correct voltage level, the correct range and the correct formula to convert the raw data from the sensor to temperature measurement.
Once the system is set up and running, the temperature can be read and displayed on the connected display device. The temperature data can also be sent to a computer, a mobile device or a cloud service using communication protocols such as USB, serial, I2C or WiFi.
In this tutorial we will see how to read the temperature measured by the DTH11 sensor and display it on a TM1637 display with Arduino.
Arduino UNO
The Arduino UNO is a microcontroller board based on the ATmega328P. It has 14 digital input/output pins, 6 analog inputs, a 16 MHz quartz crystal, a USB connection, a power jack, an ICSP header, and a reset button. It is the most popular and widely used board among the Arduino boards.
The Arduino UNO can be programmed using the Arduino programming language, which is based on C++. It uses a simple and intuitive programming environment, making it easy for beginners to get started with microcontroller programming.
The Arduino UNO can be connected to various sensors and actuators to control different devices and perform different tasks. For example, it can be used to control motors, read data from sensors, display information on an LCD screen, and communicate with other devices via serial communication protocols such as I2C and SPI.
The Arduino UNO can also be powered by a USB cable or an external power supply, making it easy to use in a wide range of projects and applications. It’s compatible with a wide range of shields (expansion boards) that adds functionality to the board, such as Ethernet, WiFi, and Bluetooth, and it’s widely supported by a strong and active community, which provides a lot of tutorials, examples and libraries to help users to get the most of the board.
TM1637 display
The TM1637 display is a type of LED display module that can be used to display digits and some characters. It is a 4-digit 7-segment LED display that can be controlled by a microcontroller, such as an Arduino. The TM1637 display module typically includes a LED driver IC, a key scan IC, and a common anode 4-digit 7-segment LED display.
The TM1637 display module has four pins: VCC, GND, DIO, and CLK. The VCC and GND pins are connected to a power source (usually 3.3V or 5V), the DIO pin is a bidirectional data pin that is connected to a digital I/O pin on the microcontroller, and the CLK pin is connected to a digital I/O pin on the microcontroller.
The TM1637 display can be controlled by using a library that provides functions to set the brightness, set the display content, and control the display. The library can be downloaded from the internet, and it can be installed in the Arduino development environment.
The TM1637 display can be used for a variety of applications, such as displaying the time, temperature, or other numerical data. It can also be used as a part of the stopwatch, where the elapsed time can be displayed on the 4-digit 7-segment LED display
DHT11 sensor
The DHT11 sensor is a temperature and humidity sensor that can be used to measure the ambient temperature and humidity. It is a low-cost and easy-to-use sensor that can be connected to an Arduino or other microcontroller. The DHT11 sensor has three pins: VCC, GND, and data. The VCC pin is connected to a power source (usually 3.3V or 5V), the GND pin is connected to ground, and the data pin is connected to a digital I/O pin on the microcontroller.
The DHT11 sensor uses a one-wire communication protocol to send the temperature and humidity data to the microcontroller. To read the data from the sensor, a library can be used that provides functions to read the data and convert it into temperature and humidity values.
The DHT11 sensor is relatively accurate and can measure temperature and humidity within a range of 0-50°C and 20-90% respectively, but it’s not recommended for precision measurements. It has a resolution of 1°C for temperature and 1% for humidity.
The basic steps to measure temperature and humidity with a DHT11 sensor and Arduino are:
connecting wires
Connecting wires are used to connect various components in an electronic circuit. They allow for the transfer of electricity, data, or signals between different devices and components.
When connecting wires to an Arduino or other microcontroller, it is important to pay attention to the correct pinout. The pinout refers to the arrangement of pins on the microcontroller and the corresponding function of each pin. The Arduino pinout can be found in the documentation provided by the manufacturer, or in various resources available online.
test plate
A test plate, also known as a test jig, is a device used to test electronic circuits and components. It is a board or plate that has been designed to hold and connect various components and devices in a specific configuration, allowing for the easy testing and measurement of their performance.
A test plate can be used to test various types of electronic circuits and components, such as microcontrollers, sensors, and actuators. It typically includes connectors and sockets for connecting wires, power supply and measurement devices such as multimeters, oscilloscopes, and power supplies.
To make the assembly, we can connect :
Here is the program that reads the temperature measured by the DTH11 sensor and displays it on a TM1637 display:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 |
#include <dht11.h> #include <TM1637Display.h> #define DHT11PIN 1 // broche DATA -> pin 1 dht11 DHT11; // Module connection pins (Digital Pins) #define CLK 2 #define DIO 3 TM1637Display display(CLK, DIO); void setup() { display.setBrightness(0x0f); } void loop() { DHT11.read(DHT11PIN); display.showNumberDec((float)DHT11.temperature); // Display Temperature in TM1637 Display } |